We have more to share with you. Follow us along to modal 2
That's everything!
Your inbox is getting full, would you like us to enable automatic archiving of old messages?
Are you sure you want to delete your account
Are you sure you want to delete your account
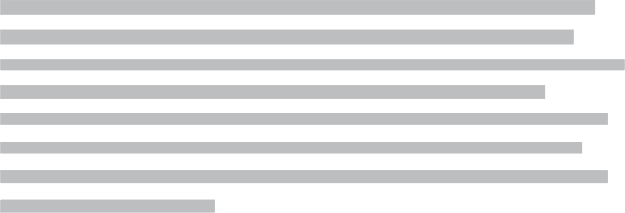
Are you sure you want to delete your account
Are you sure you want to delete your account
Give us your feedback
Do you want to change that thing to something else?
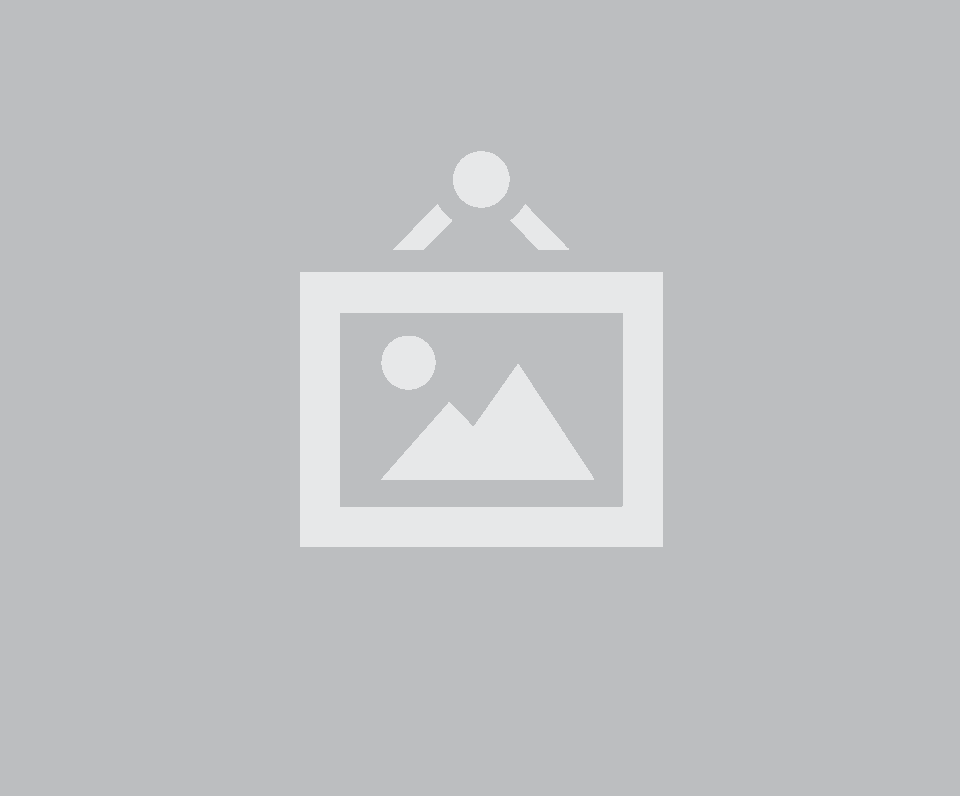
This is an example of expanded content that will cause the modal's content to scroll
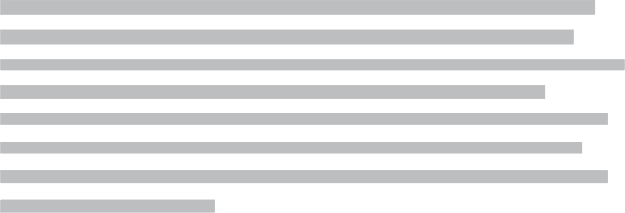
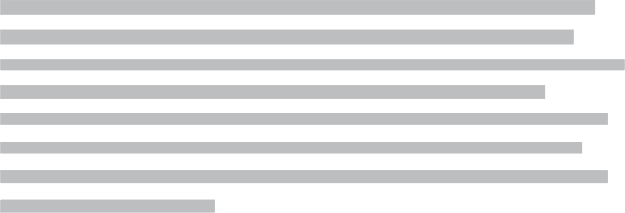
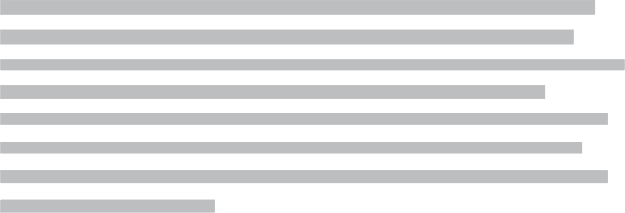
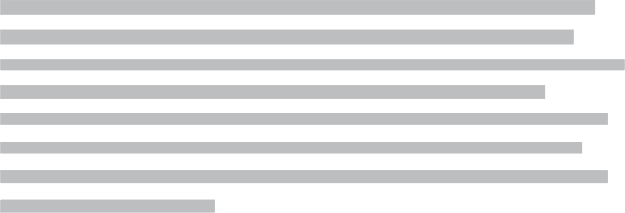
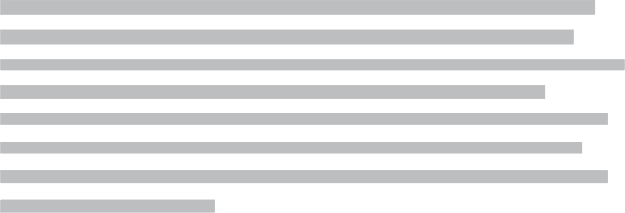
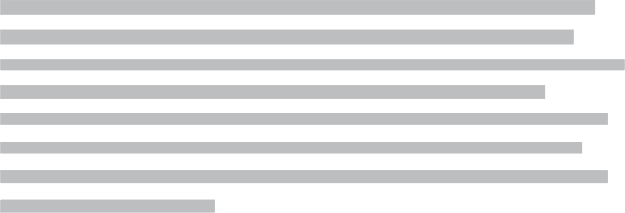
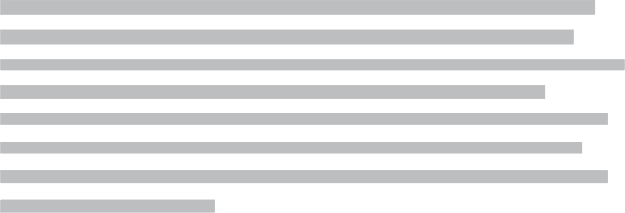
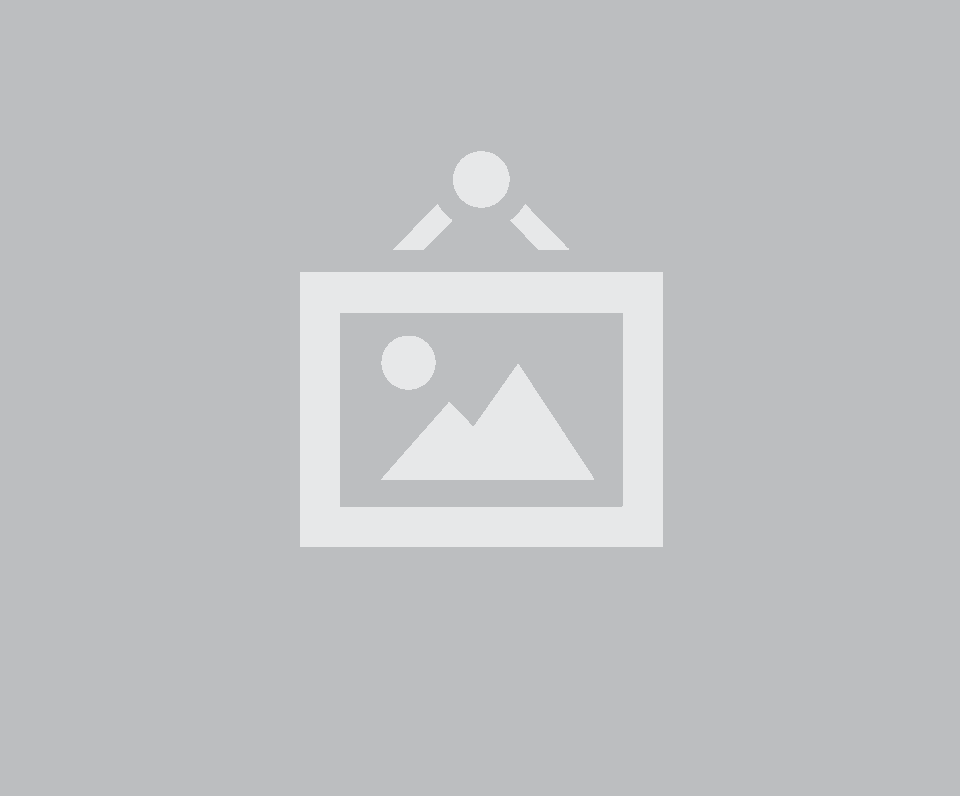
This is an example of expanded content that will cause the modal's dimmer to scroll
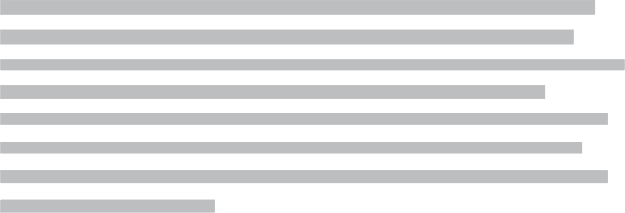
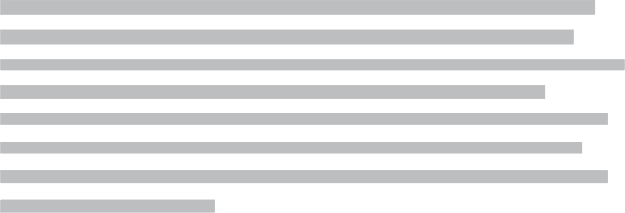
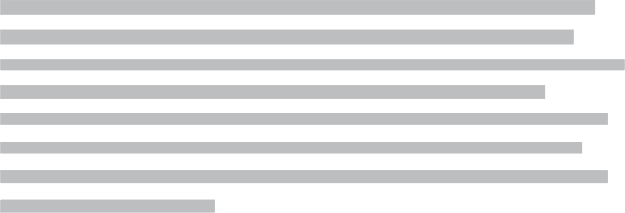
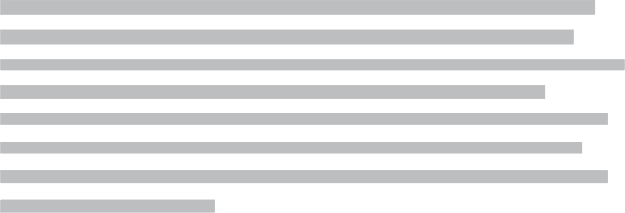
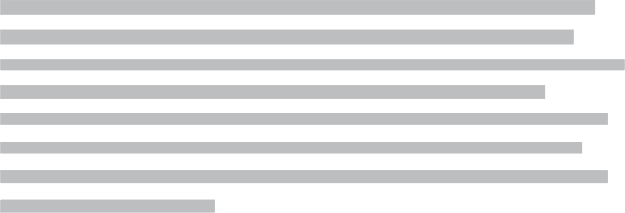
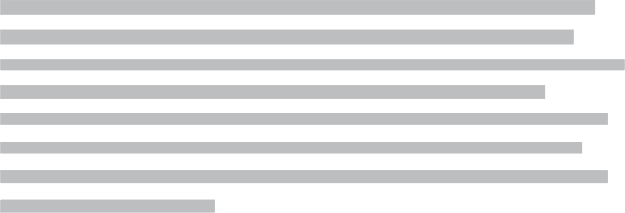
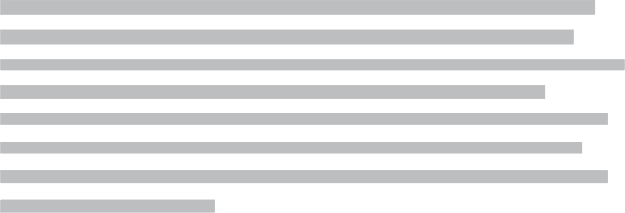
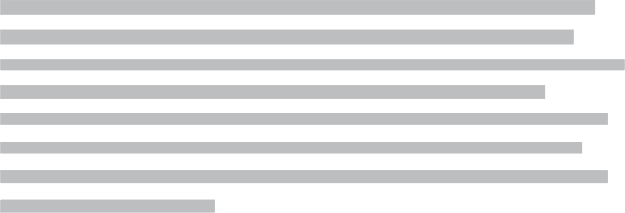
Content is centered
They are aligned to the left
Basic actions below
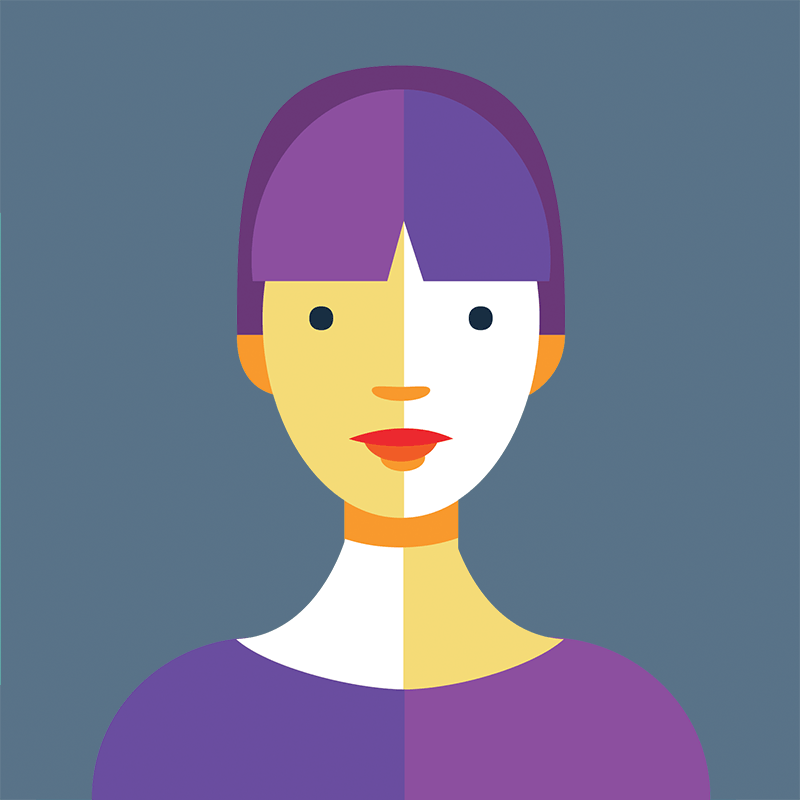
We've found the following gravatar image associated with your e-mail address.
Is it okay to use this photo?
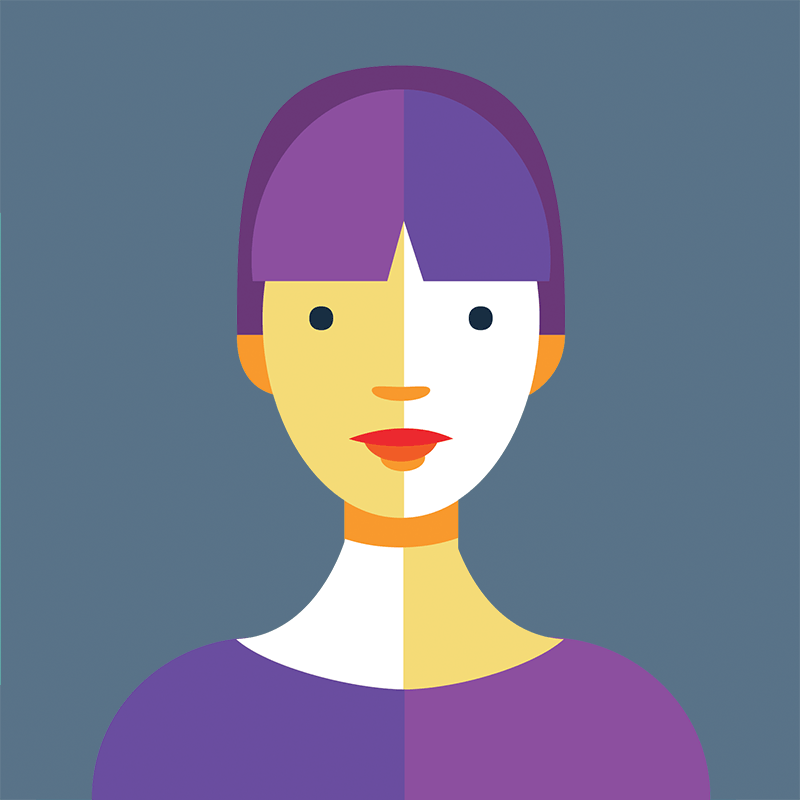
We've found the following gravatar image associated with your e-mail address.
Is it okay to use this photo?
Types
Modal
A standard modal
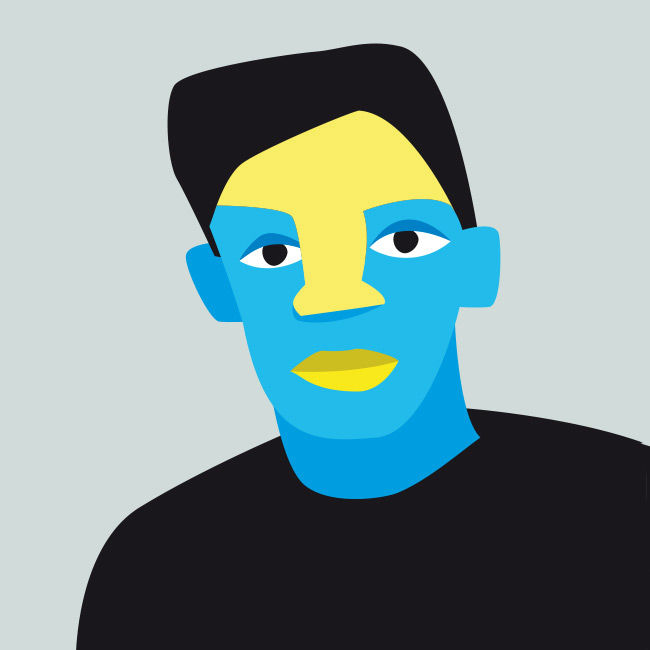
We've grabbed the following image from the gravatar image associated with your registered e-mail address.
Is it okay to use this photo?
Basic
A modal can reduce its complexity
Your inbox is getting full, would you like us to enable automatic archiving of old messages?
Content
Header
A modal can have a header
Content
A modal can contain content
Image Content
A modal can contain image content
Actions
A modal can contain a row of actions
Variations
Full Screen
A modal can use the entire size of the screen
Overlay Full Screen
A modal can overlay the entire screen
Size
A modal can vary in size
Inverted
A modal can be shown inverted on white dimmer...
... or default black dimmer
Scrolling Content
A modal can have a scrolling content
Very long content goes here
Center Aligned
You can center align the header, the content or even actions individually
Content is centered
Left Actions
You can also place the action buttons to the left
They are aligned to the left
Basic Header and Actions
Header and/or Actions can also appear on the same basic background as the content
Basic Actions below
States
Active
An active modal is visible on the page
Examples
Disabling Vertical Centering
When your modal has dynamic content, or multiple steps, it can make sense to disable centering so content doesn't jump around vertically when its height changes.
Top or Bottom aligned
If you leave the centered
option to the default true
, you can also force positioning of the modal by adding additional classes top aligned
(which equals to the usage of centered:false
) or bottom aligned
to the modal itself
Scrolling Modal
When your modal content exceeds the height of the browser the scrollable area will automatically expand to include just enough space for scrolling, without scrolling the page below.
Internally Scrolling Content
You may prefer to have the content of your modal scroll itself, you can do this by using the scrolling content
variation.
Multiple Modals
A modal can open a second modal. If you use allowMultiple: true
parameter the second modal will be opened on top of the first modal. Otherwise the modal will be closed before the second modal is opened.
Forcing a Choice
You can disable a modal's dimmer from being closed by click to force a user to make a choice
Approve / Deny Callbacks
Modals will automatically tie approve deny callbacks to any positive/approve, negative/deny or ok/cancel buttons.
Attach events
A modal can attach events to another element
Transitions
A modal can use any named ui transition.
Dimmer Variations
Modals can specify additional variations like blurring
or inverted
which adjust how the dimmer displays.
Initializing a modal
Via Javascript properties
You can create temporary modals without the need to create markup on your own. Temporary modals will be removed from the DOM once closed by default if there isn't a custom onHidden
callback given.
Via existing DOM node
A modal can be included anywhere on the page. On initialization a modal's current size will be cached, and the element will be detached from the DOM and moved inside a dimmer.
Reuse existing modal with new content
You can prepare a modal markup as a general template and reuse it's general style but provide actual content via js properties.
'+Math.random().toString(16).substr(2).toUpperCase()+'', classContent: 'centered', class: 'small' }).modal('show');
Behavior
All the following behaviors can be called using the syntax:
Behavior | Description |
---|---|
show | Shows the modal |
hide | Hides the modal |
toggle | Toggles the modal |
refresh | Refreshes centering of modal on page |
show dimmer | Shows associated page dimmer |
hide dimmer | Hides associated page dimmer |
hide others | Hides all modals not selected modal in a dimmer |
hide all | Hides all visible modals in the same dimmer |
cache sizes | Caches current modal size |
can fit | Returns whether the modal can fit on the page |
is active | Returns whether the modal is active |
set active | Sets modal to active |
destroy | Destroys instance and removes all events |
Config Templates
A config template is a special behavior to immediately show preconfigured temporary modals. Three basic templates are included: alert
, confirm
, prompt
as equivalents to existing vanilla JS variants, but with more possibilities to customize the look & feel.
Alert
Possible parameters are: title, content, handler (in that order to stay nearly identical to vanilla js usage) or a given object {title:'',content:'',handler:function(){}}
where as title and content can contain HTML.
Confirm
The parameter list and logic is the same as for alert. The selected boolean choice will be provided to a given callback handler.
Prompt
The call for prompt is basically identical to alert and confirm. There are 2 more options available when an object is given placeholder
and defaultValue
.
If you provide HTML Code for the content and this contains an input, this will be used as the inputfield. Otherwise it creates one dynamically for you.
Create your own template
By extending the modals templates object once, you can define your own custom config templates. It has to return an object which will be merged into the modals settings prior to creating/showing the modal.
is the best!', class: 'inverted', classContent: 'centered', dimmerSettings: { variation: 'inverted' } } }
Reuse this whenever you need
Settings
Modal Settings
Modal settings modify the modal's behavior
Setting | Default | Description |
---|---|---|
detachable | true | If set to false will prevent the modal from being moved to inside the dimmer |
useFlex | 'auto' | Auto will automatically use flex in browsers that support absolutely positioned elements inside flex containers. Setting to true/false will force this setting for all browsers. |
autofocus | true | When true, the first form input inside the modal will receive focus when shown. Set this to false to prevent this behavior. |
restoreFocus | true | When false, the last focused element, before the modal was shown, will not get refocused again when the modal hides. This could prevent unwanted scrolling behaviors after closing a modal. |
autoShow | false | When true, immediately shows the modal at instantiation time. |
observeChanges | false | Whether any change in modal DOM should automatically refresh cached positions |
allowMultiple | false | If set to true will not close other visible modals when opening a new one |
inverted | false | If inverted dimmer should be used |
blurring | false | If dimmer should blur background |
centered | true | If modal should be center aligned |
keyboardShortcuts | true | Whether to automatically bind keyboard shortcuts. This will close the modal when the ESC-Key is pressed. |
offset | 0 | A vertical offset to allow for content outside of modal, for example a close button, to be centered. |
context | body | Selector or jquery object specifying the area to dim |
closable | true | Setting to false will not allow you to close the modal by clicking on the dimmer |
dimmerSettings |
{
closable : false,
useCSS : true
}
|
You can specify custom settings to extend UI dimmer |
transition | scale | Named transition to use when animating menu in and out, full list can be found in ui transitions docs. Alternatively you can provide an object to set individual values for hide/show transitions as well as hide/show duration.
{
showMethod : 'fade',
showDuration : 200,
hideMethod : 'zoom,
hideDuration : 500,
}
|
duration | 400 | Duration of animation. The value will be ignored when individual hide/show duration values are provided via the transition setting |
queue | false | Whether additional animations should queue |
scrollbarWidth | 10 | Used internally to determine if the webkit custom scrollbar was clicked to prevent hiding the dimmer. This should be set to the same (numeric) value as defined for @customScrollbarWidth in site.less in case you are using a different theme |
Callbacks
Callbacks specify a function to occur after a specific behavior.
Setting | Context | Description |
---|---|---|
onShow | Modal | Is called when a modal starts to show. If the function returns false , the modal will not be shown. |
onVisible | Modal | Is called after a modal has finished showing animating. |
onHide($element) | Modal | Is called after a modal starts to hide. If the function returns false , the modal will not hide. |
onHidden | Modal | Is called after a modal has finished hiding animation. |
onApprove($element) | Click | Is called after a positive, approve or ok button is pressed. If the function returns false , the modal will not hide. |
onDeny($element) | Modal | Is called after a negative, deny or cancel button is pressed. If the function returns false the modal will not hide. |
DOM Settings
DOM settings specify how this module should interface with the DOM
Setting | Default | Description |
---|---|---|
namespace | modal | Event namespace. Makes sure module teardown does not effect other events attached to an element. |
selector |
selector : {
title : '> .header',
content : '> .content',
actions : '> .actions',
close : '> .close',
approve : '.actions .positive, .actions .approve, .actions .ok',
deny : '.actions .negative, .actions .deny, .actions .cancel',
dimmer : '> .ui.dimmer',
bodyFixed: '> .ui.fixed.menu, > .ui.right.toast-container, > .ui.right.sidebar, > .ui.fixed.nag, > .ui.fixed.nag > .close',
prompt : '.ui.input > input'
}
|
|
className |
className : {
active : 'active',
animating : 'animating',
blurring : 'blurring',
inverted : 'inverted',
legacy : 'legacy',
loading : 'loading',
scrolling : 'scrolling',
undetached : 'undetached',
front : 'front',
close : 'close icon',
button : 'ui button',
modal : 'ui modal',
title : 'header',
content : 'content',
actions : 'actions',
template : 'ui tiny modal',
ok : 'positive',
cancel : 'negative',
prompt : 'ui fluid input',
innerDimmer: 'ui inverted dimmer'
}
|
Config Template Settings
Config Template Settings define default content for dynamically created modals
Setting | Default | Description |
---|---|---|
title | '' | Content of the modal header |
content | '' | Content of the modal content |
closeIcon | false | Whether the modal should include a close icon |
actions | false | An array of objects. Each object defines an action with properties text ,class ,icon and click .
actions: [{
text : 'Wait',
class : 'red',
icon : 'exclamation',
click : function(){}
}]
|
preserveHTML | true | Whether HTML included in given title, content or actions should be preserved. Set to false in case you work with untrusted 3rd party content |
class | '' | Can hold a string to be added to the modal class to control its appearance. |
classTitle | '' | Can hold a string to be added to the title class to control its appearance. |
classContent | '' | Can hold a string to be added to the content class to control its appearance. |
classActions | '' | Can hold a string to be added to the actions class to control its appearance. |
fields |
fields : {
class : 'class',
text : 'text',
icon : 'icon',
click : 'click'
}
|
|
text |
text: {
ok : 'Ok',
cancel: 'Cancel'
}
|
Debug Settings
Debug settings controls debug output to the console
Setting | Default | Description |
---|---|---|
name | Modal | Name used in debug logs |
silent | false | Silences all console output including error messages, regardless of other debug settings. |
debug | false | Provides standard debug output to console |
performance | true | Provides standard debug output to console |
verbose | false | Provides ancillary debug output to console |
error |
error : {
dimmer : 'UI Dimmer, a required component is not included in this page',
method : 'The method you called is not defined.',
notFound : 'The element you specified could not be found'
}
|