Types
Minimal
A minimal toast will just display a message.
Titled
You can add a title to your toast.
Progress Bar
You can attach a progress bar to your toast.
The progress bar can have its own individual color
Variations
Toast Type
A toast can be used to display different types of informations.
Position
A toast can appear at different positions on the screen.
Attached Position
A toast can have an attached position which will show the toast over the whole width of the screen. Just like notifications on mobile devices.
Direction
Toasts can stack horizontal
Center Aligned
The toasts content can be shown center aligned.
Duration
You can choose how long a toast should be displayed.
You can even avoid a toast to disappear.
Setting the value to auto
calculates the display time by the amount of containing words
You can adjust the calculation by modifying the default values for minDisplayTime and wordsPerMinute' }) ;
Use Message Style
You can use all of the styles and colors from the message component
Increasing Progress Bar
The progress bar could be be raised instead of lowered
Color Variants
You can use all of the usual color names
Inverted Colors
Same as above, just add inverted
to the class definition
Actions
General
Define click actions to your toast by providing a text and/or icon, optional class and click handler.
Basic
The classActions
setting provides you a way to adjust the overall appearance of the action buttons. Using basic
class does not lighten the actions background. left
aligns the buttons to the left
Centered
The actions buttons can also be shown centered
Attached
Using attached
converts your actions into a Button group. Also add top
to display the actions attached to the top of the toast
Vertical
You can use vertical
to display your actions to the right of the toast.
Vertical attached
Vertical actions can also be displayed as button groups using vertical attached
Vertical attached actions also support left
Examples
Without icon
You can choose to hide the status icon.
Individual icon
Use whatever you like from the included FontAwesome gallery
Image
Provide an image path to display it just like the icon does.
Adjust the image via the separate classImage
setting
The image can be shown without any padding by providing the image
class New in 2.9.0
Close Icon
You can force the user to click a close Icon instead of clicking anywhere on the toast to close it
The close icon can also be displayed to the left New in 2.8.0
Transitions
You can set other transitions types and durations.
Actions and centered and attached position
Using actions in attached position variants in combination with centered toast content does only support attached actions. This is because of the flexbox usage to align a possible icon/image next to the content.
Create from DOM
By creating your toasts out of existing DOM nodes you can make use of other existing FUI components
Toast
Template
You can reuse a dom template and provide its content via setting parameters
Approve / Deny Callbacks
Just like Modal, Toast will automatically tie approve/deny callbacks to any positive/approve, negative/deny or ok/cancel buttons without the need to create actions via setting
Message
Card
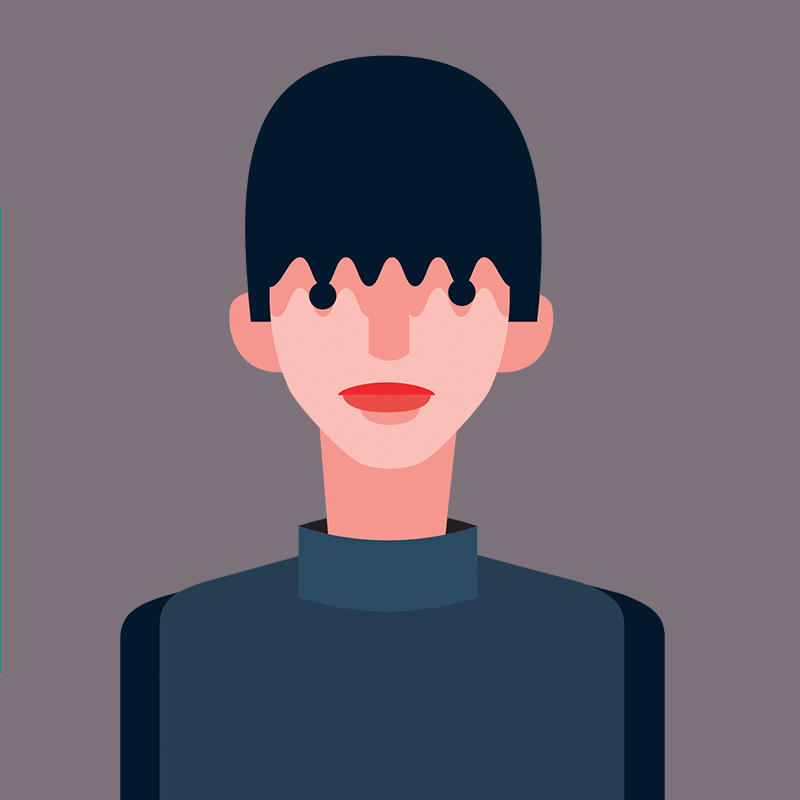
Interaction
Prevent pausing
The default behavior when hovering over the toast is to pause decreasing of the display time.
You can force the toast to not pause by setting pauseOnHover
to false
Prevent closing
The default behavior when clicking on a toast is to close it.
You can force the toast to not do this by setting closeOnClick
to false
Access created toast
As each call to .toast()
returns the instance of the created toast, you are able to interact with the displayed toast via javascript behaviors
Appearance
Migration from 2.7.0
The toast defaults changed in 2.8.0. In case you want to keep the old defaults for all of your toasts, override the defaults as follows
General HTML Layout
The general layout of a rendered toast might be useful for people who want to implement their own javascript display logic and just want to reuse Fomantics HTML CSS Layout.
The following structure is used to position the toasts. Each position container implements a toast-box
per toast. The extra wrapper is needed to make dynamic elements like progressbar independent of the toast layout.
A single toast layout inside the above mentioned toast-box
has the following structure

Behaviors
All the following behaviors can be called using the syntax:
Behavior | Description |
---|---|
animate pause | Pauses the display time decrease (and possible progress bar animation) |
animate continue | Continues decreasing display time (and possible progress bar animation) |
close | Closes the toast |
get toasts | Returns all toasts as an array of objects which are visible within the current toast-container |
get remainingTime | Returns the remaining time in milliseconds |
destroy | Destroys instance and removes all events |
Settings
Toast Settings
Settings to configure toast behavior
Setting | Default | Description |
---|---|---|
position | top right | Sets where the toast can be displayed. Can be top right , top center , top left , bottom right , bottom center , bottom left and centered |
horizontal | false | If the toasts should stack horizontal instead of vertical |
class | neutral | Define the class of notification. Can be any existing color definition or info , success , warning and error . If ui message is used in className.toast option (see below), this option can hold any supported class of the message component |
classProgress | false | Can hold a string to be added to the progress bar class, for example a separate color |
classActions | false | Can hold a string to be added to the actions class to control its appearance. Usually a combination of basic , left , top , bottom , vertical and attached |
classImage | mini | Can hold a string to be added to the image class. mini , tiny , small and avatar are supported out of the box |
context | body | Selector or jquery object specifying the area to attach the toast container to |
displayTime | 3000 | Set the time (in ms) of the toast appearance. Set 0 to disable the automatic dismissal. Set auto to calculate the time by the given amount of words within the toast |
minDisplayTime | 1000 | Minimum display time in case displayTime is set to 'auto' |
wordsPerMinute | 120 | Base to calculate display time in case displayTime is set to 'auto' |
showImage | false | If an URL to an image is given, that image will be shown to the left of the toast |
alt | false | If showImage is true, the alt setting can provide a string used for the image alt attribute |
showIcon | true | Define if the toast should display an icon which matches to a given class. If a string is given, this will be used as icon classname |
closeIcon | false | This will make the toast closable by the top right corner icon instead of clicking anywhere on the toast when set to true . When set to left the closeIcon is shown to the left instead of right |
closeOnClick | true | Set to false to avoid closing the toast when it is clicked |
cloneModule | true | If a given DOM-Node should stay reusable by using a clone of it as toast. If set to false the original DOM-Node will be detached and removed from the DOM then the toast is closed |
showProgress | false | Displays a progress bar on top or bottom increasing until displayTime is reached. . false won't display any progress bar. If displayTime option is 0, this option is ignored |
progressUp | false | true Increases the progress bar from 0% to 100%false Decreases the progress bar from 100% to 0% |
pauseOnHover | true | Set to false if the display timer should not pause when the toast is hovered |
compact | true | true will display the toast in a fixed width, false displays the toast responsively with dynamic width |
opacity | 1 | Opacity Value of the toast after the show-transition |
newestOnTop | false | Define if new toasts should be displayed above the others |
preserveHTML | true | Whether HTML included in given title, message or actions should be preserved. Set to false in case you work with untrusted 3rd party content |
transition |
transition: {
showMethod : 'scale',
showDuration : 500,
hideMethod : 'scale',
hideDuration : 500,
closeEasing : 'easeOutCubic',
closeDuration: 500,
}
|
Settings to set the transitions and durations during the show or the hide of a toast |
Callbacks
Callbacks specify a function to occur after a specific behavior.
Parameters | Description | |
---|---|---|
onShow | $module | Callback before toast is shown. Returning false from this callback will cancel the toast from showing. |
onVisible | $module | Callback after toast is shown. |
onClick | $module | Callback after popup is clicked in. |
onHide | $module | Callback before toast is hidden. Returning false from this callback will cancel the toast from hiding. |
onHidden | $module | Callback after toast is hidden. |
onRemove | $module | Callback before toast is destroyed. |
onApprove | $module | Callback when an existing button with class positive or ok or approve is clicked. Return false to avoid closing the toast |
onDeny | $module | Callback when an existing button with class negative or cancel or deny is clicked. Return false to avoid closing the toast |
Content Settings
Settings to specify toast contents
Setting | Description |
---|---|
title | A title for the toast. Leave empty to not display it |
message | Message to display |
actions | An array of objects. Each object defines an action with properties text ,class ,icon and click
actions: [{
text : 'Wait',
class : 'red',
icon : 'exclamation',
click : function(){}
}]
|
DOM Settings
DOM settings specify how this module should interface with the DOM
Setting | Default | Description |
---|---|---|
namespace | toast | Event namespace. Makes sure module teardown does not effect other events attached to an element. |
selector |
selector : {
container : '.ui.toast-container',
box : '.toast-box',
toast : '.ui.toast',
title : '.header',
message : '.message:not(.ui)',
image : '> img.image, > .image > img',
icon : '> i.icon',
input : 'input:not([type="hidden"]), textarea, select, button, .ui.button, ui.dropdown',
clickable : 'a, details, .ui.accordion',
approve : '.actions .positive, .actions .approve, .actions .ok',
deny : '.actions .negative, .actions .deny, .actions .cancel'
}
|
DOM Selectors used internally |
className |
className : {
container : 'ui toast-container',
absolute : 'absolute',
box : 'floating toast-box',
progress : 'ui attached active progress',
toast : 'ui toast',
icon : 'centered icon',
visible : 'visible',
content : 'content',
title : 'ui header',
message : 'message',
actions : 'actions',
extraContent : 'extra content',
button : 'ui button',
buttons : 'ui buttons',
close : 'close icon',
image : 'ui image',
vertical : 'vertical',
horizontal : 'horizontal',
attached : 'attached',
inverted : 'inverted',
compact : 'compact',
pausable : 'pausable',
progressing : 'progressing',
top : 'top',
bottom : 'bottom',
left : 'left',
basic : 'basic',
unclickable : 'unclickable',
}
|
Class names used to attach style to state |
icons |
icons : {
info : 'info',
success : 'checkmark',
warning : 'warning',
error : 'times'
}
|
Icon names used internally |
text |
text : {
close : 'Close'
}
|
Display strings |
Debug Settings
Debug settings controls debug output to the console
Setting | Default | Description |
---|---|---|
name | Toast | Name used in debug logs |
silent | false | Silences all console output including error messages, regardless of other debug settings. |
debug | false | Provides standard debug output to console |
performance | true | Provides standard debug output to console |
verbose | false | Provides ancillary debug output to console |
errors |
error: {
method : 'The method you called is not defined.',
noElement : 'This module requires ui transitions.',
verticalCard : 'Vertical but not attached actions are not supported for card layout'
}
|