Rating
Definition
Types
Rating Flexbox
A basic rating
States
Disabled
A rating can be started in non-interactive mode by adding a disabled
class
Variations
Examples
Setting existing values
The icon can be set either using metadata value data-icon
or the setting icon
.
The starting rating can be set either using metadata value data-rating
or the setting initialRating
.
The maximum rating can be be set using the metadata value data-max-rating
or the settings maxRating
, or you can just include the icon HTML yourself on initialization to avoid the overhead of the DOM template insertions
.
$('.toggle.example .rating')
.rating({
initialRating: 2,
maxRating: 4
})
;
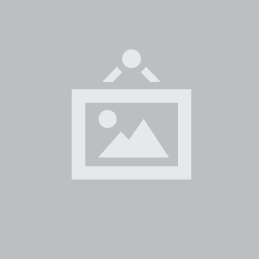
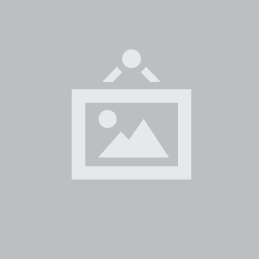
Read-Only Ratings
You can disable or enable interactive rating
Run Code$('.toggle.example .rating')
.rating('disable')
;
$('.toggle.example .rating')
.rating('enable')
;
Clearing Ratings
When clearable is set to true
you can clear the rating by clicking on the current start rating.
$('.clearing.example .rating')
.rating('setting', 'clearable', true)
;
Initializing
Metadata
You can specify the icon, starting rating and max rating in metadata.
$('.ui.rating')
.rating()
;
<div class="ui rating" data-icon="circle" data-rating="3" data-max-rating="5"></div>
Javascript
You can specify the icon and rating values in Javascript
$('.ui.rating')
.rating({
icon: 'circle',
initialRating: 3,
maxRating: 5
})
;
<div class="ui rating"></div>
Without Javascript
If you don't need user interaction, you can use the rating module as a pure read-only CSS element
<div class="ui red rating disabled">
<i class="heart icon active"></i>
<i class="heart icon active"></i>
<i class="heart icon active"></i>
<i class="heart icon"></i>
<i class="heart icon"></i>
</div>
Behaviors
All the following behaviors can be called using the syntax:
$('.ui.rating')
.rating('behavior name', argumentOne, argumentTwo)
;
Behavior | Description |
---|---|
set rating(rating) | Sets rating programmatically |
get rating | Gets current rating |
disable | Disables interactive rating mode |
enable | Enables interactive rating mode |
clear rating | Clears current rating |
destroy | Destroys instance and removes all events |
Rating Settings
Rating settings modify the rating's behavior
Setting | Default | Description |
---|---|---|
icon | star | The icon classname |
initialRating | 0 | A number representing the default rating to apply |
maxRating | 4 | The maximum rating value |
fireOnInit | false | Whether callbacks like onRate should fire immediately after initializing with the current value. |
clearable | auto | By default a rating will be only clearable if there is 1 icon. Setting to true/false will allow or disallow a user to clear their rating |
interactive | true | Whether to enable user's ability to rate |
Callbacks
Callbacks specify a function to occur after a specific behavior.
Setting | Context | Description |
---|---|---|
onRate(value) | Rating | Is called after user selects a new rating |
DOM Settings
DOM settings specify how this module should interface with the DOM
Setting | Default | Description |
---|---|---|
namespace | rating | Event namespace. Makes sure module teardown does not effect other events attached to an element. |
selector |
|
|
className |
|
Debug Settings
Debug settings controls debug output to the console
Setting | Default | Description |
---|---|---|
name | Rating | Name used in debug logs |
silent | false | Silences all console output including error messages, regardless of other debug settings. |
debug | false | Provides standard debug output to console |
performance | true | Provides standard debug output to console |
verbose | false | Provides ancillary debug output to console |
error |
|