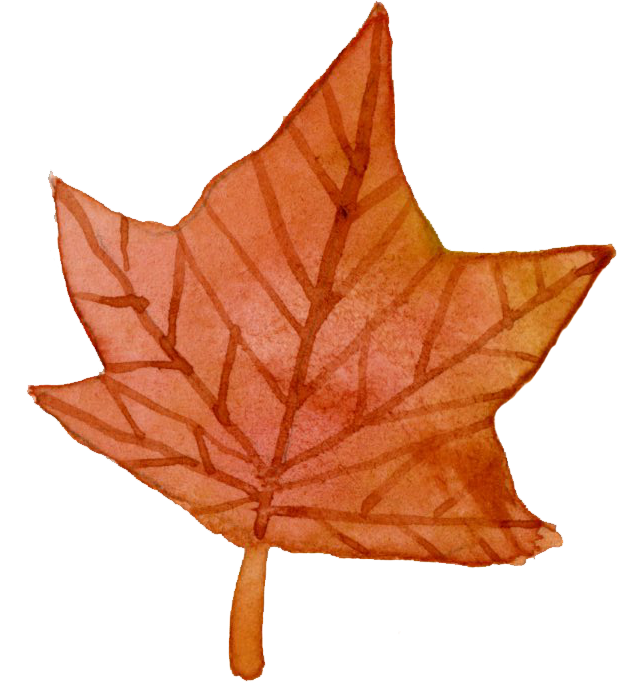
Transitions
Scale
An element can scale into or out of view
Zoom
An element can zoom into view from far away
Fade
An element can fade into or out of view descending and ascending
Flip
An element can flip into or out of view vertically or horizontally
Drop
An element can drop into view from above
Fly
An element can fly in from off canvas
Swing
An element can swing into view
Browse
An element can appear and disappear as part of a series
Slide
An element can appear to slide in from above or below
Static Animations
Pulsating
An element can have pulsating edges. This works best on circular or square elements.
Jiggle
An element can jiggle to draw attention to its shape
Flash
An element can flash to draw attention to itself
Shake
An element can shake to draw attention to its position
Pulse
An element can pulse to draw attention to its visibility
Tada
An element can give user positive feedback for an action
Bounce
An element can bounce to politely remind you of itself
Glow
An element can glow to show its position in a page.
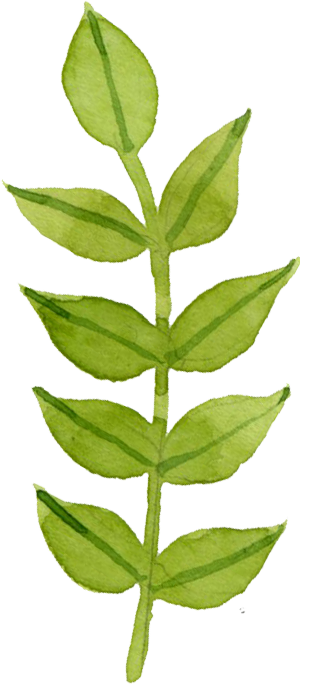
Visibility
After the animation queue finishes for an element, its final visibility state is determined. If an animation is an outward transition, the final visibility status will be hidden. If an animation is inward the element will be visible after the animation finishes.
Specifying a direction
To force an animation direction, add either in
or out
to the animation name.
Automatic Direction
If an animation direction is not specified it will automatically be determined based on the elements current visibility. For example, if the element is visible the animation "fade out" will be called, if the animation is not visible "fade in".
Grouped Transitions
Animation Intervals
When animating several different items in a group you may want to use an interval so that each item appears consecutively
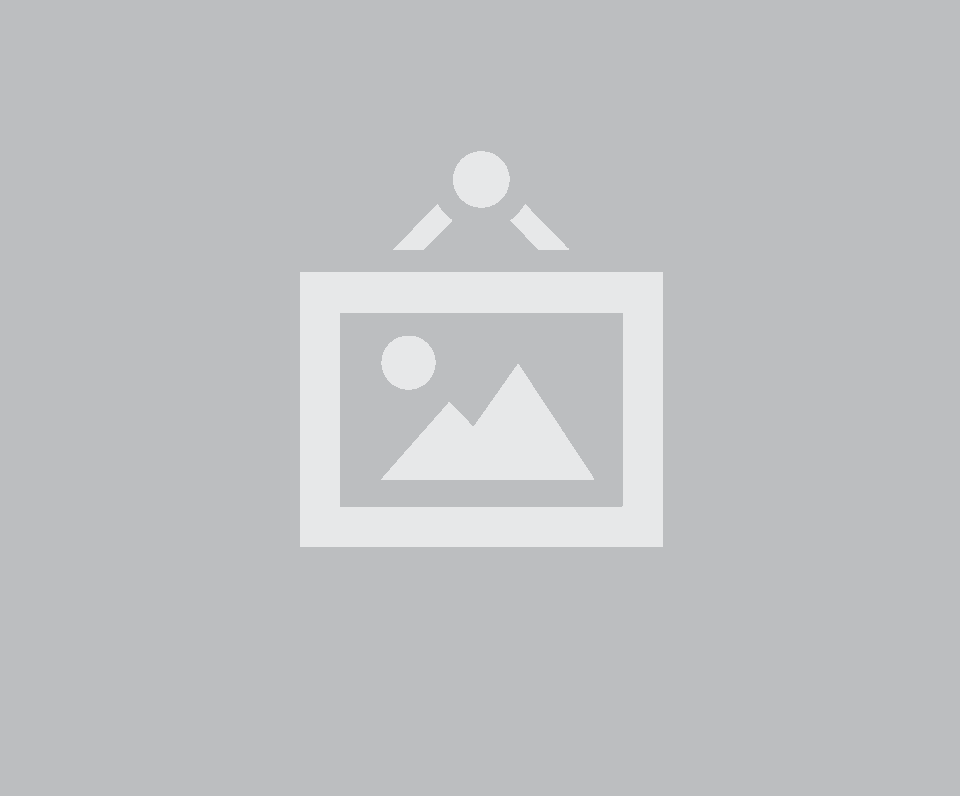
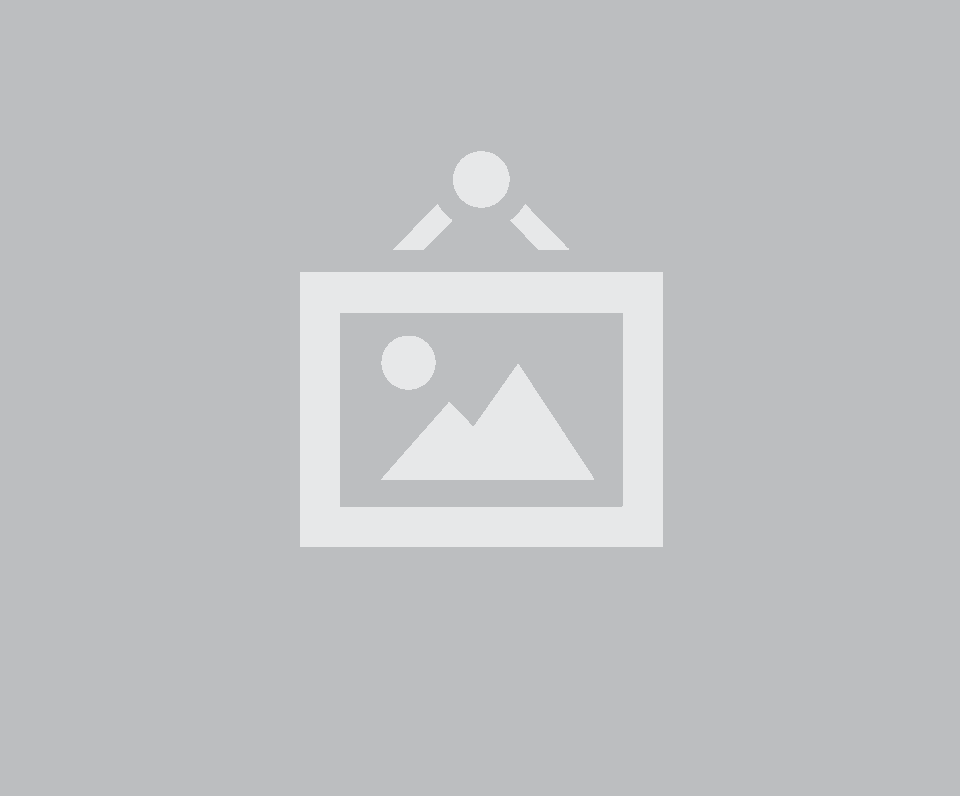
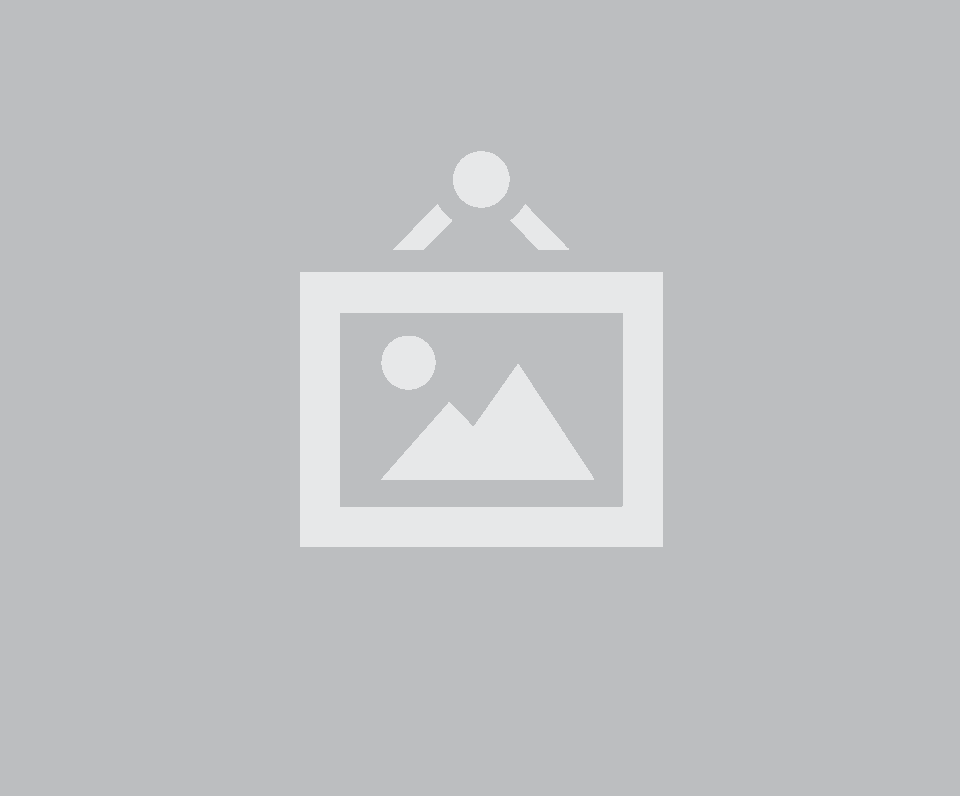
Animation Order
When hiding groups of elements, the default setting uses reverse: 'auto'
. This will automatically reverse the order of animations from back-to-front when hiding elements to avoid layout reflows. When showing elements the order will return to its normal sequence.
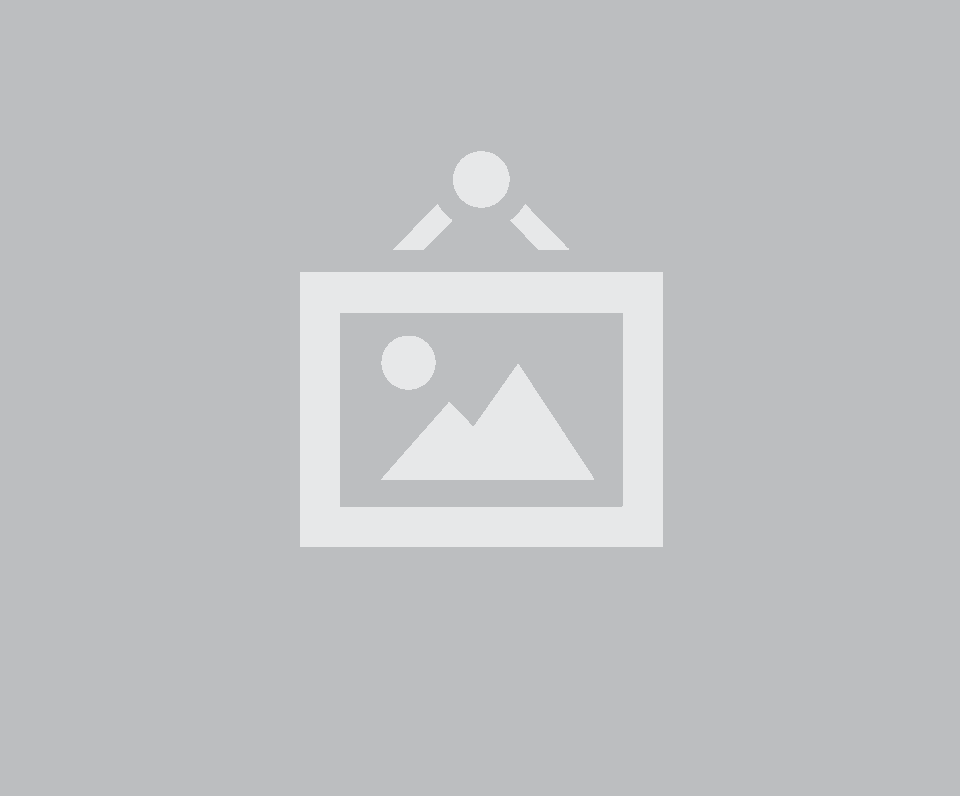
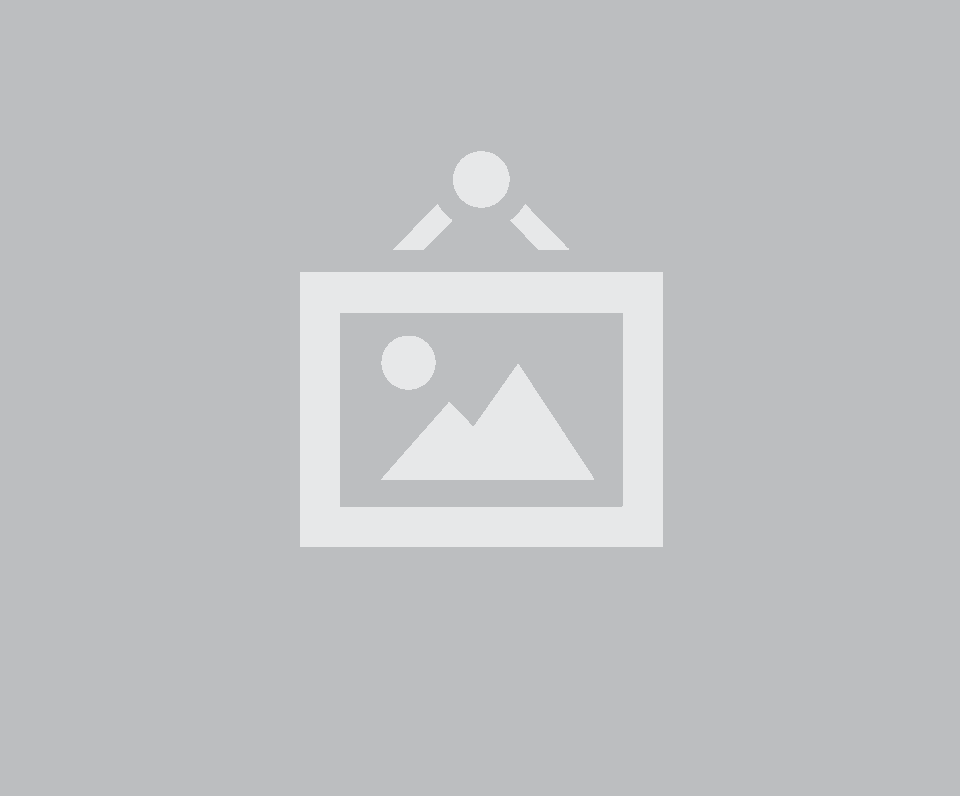
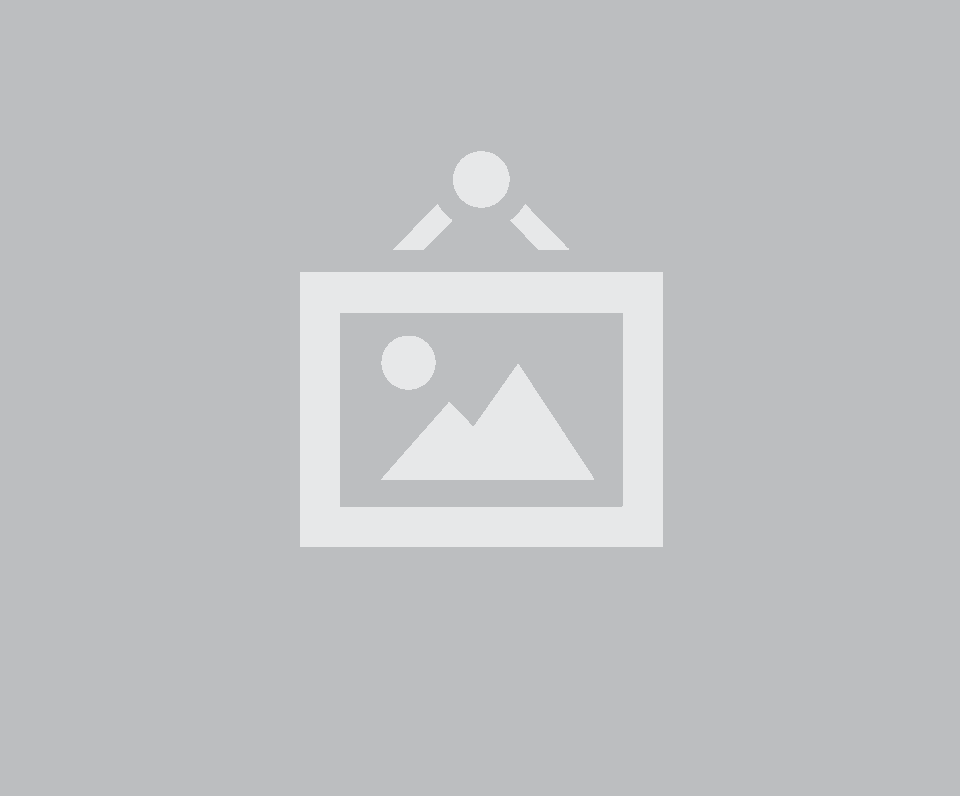
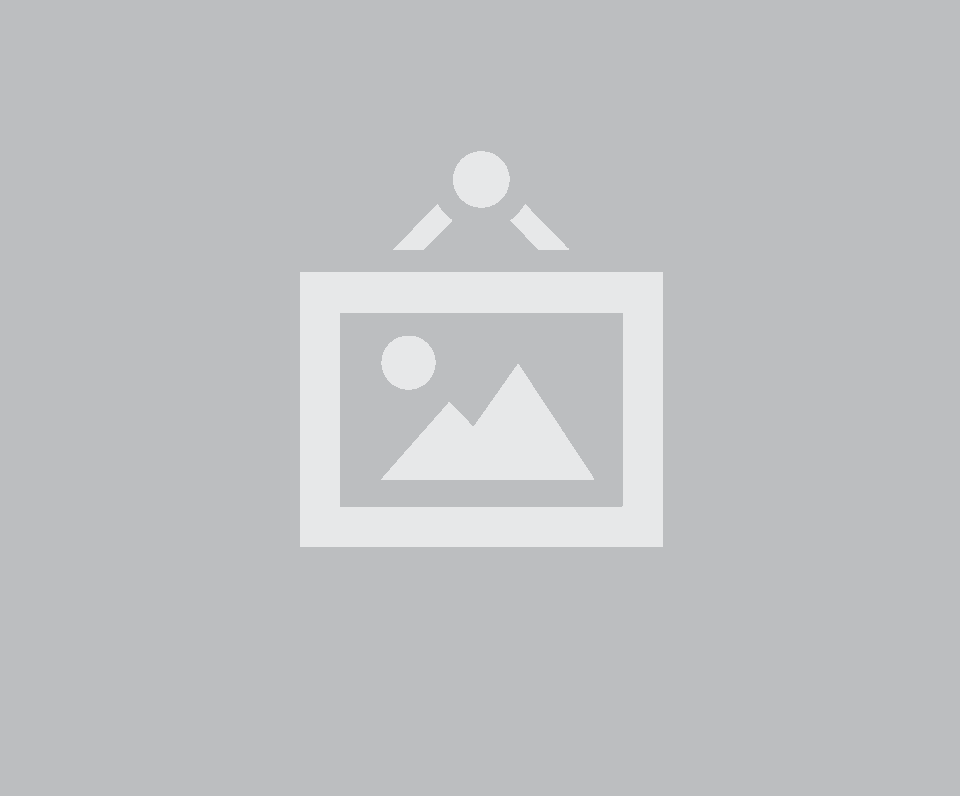
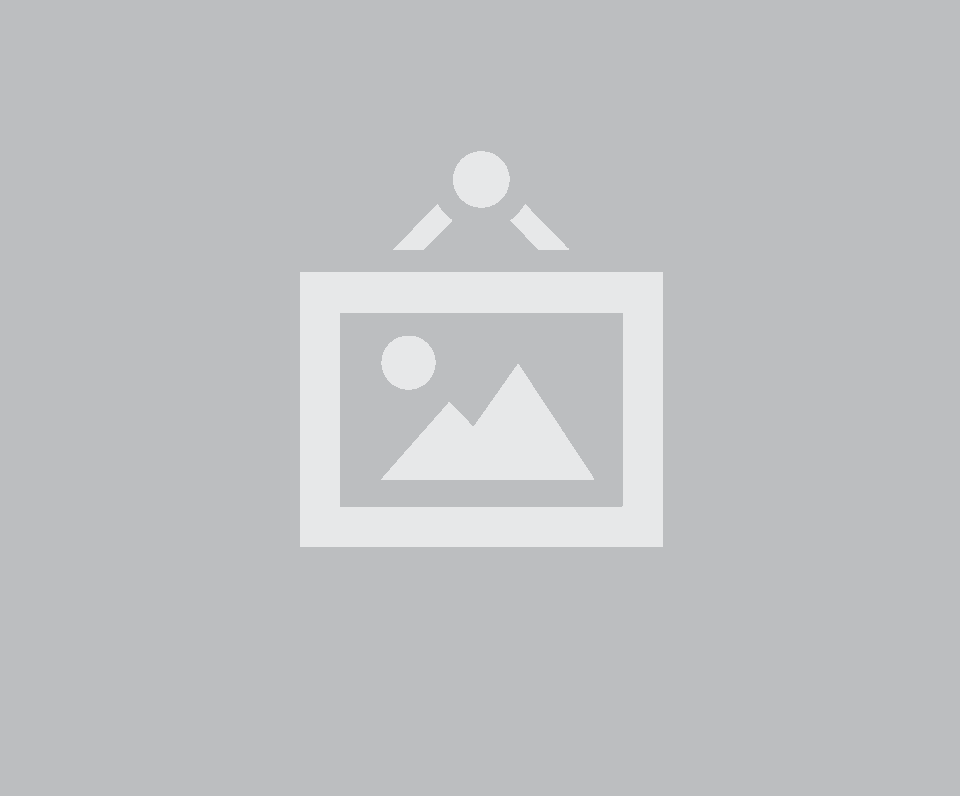
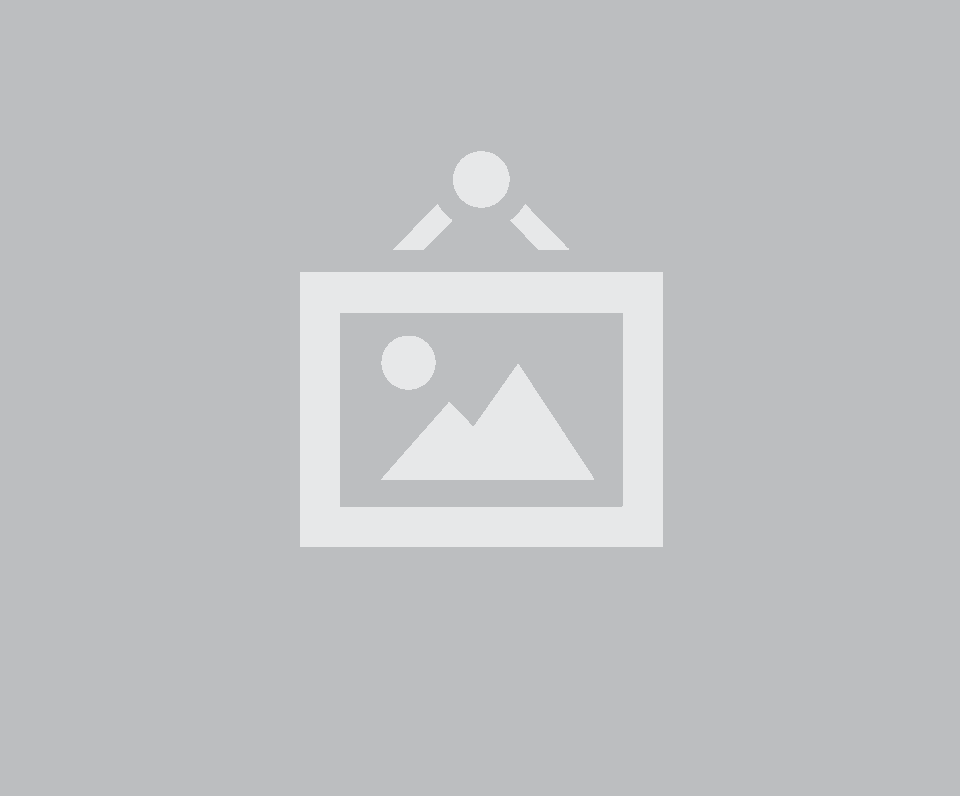
Forcing Order
If you need to manually force reverse animations regardless of the animation direction, you can use reverse: true
.
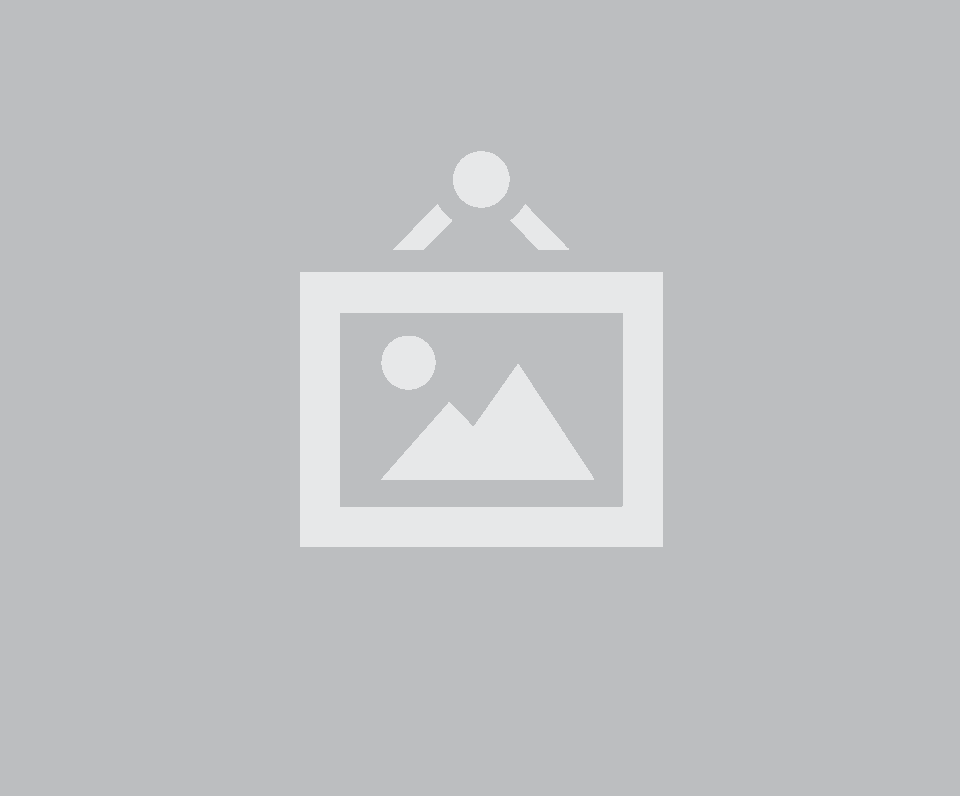
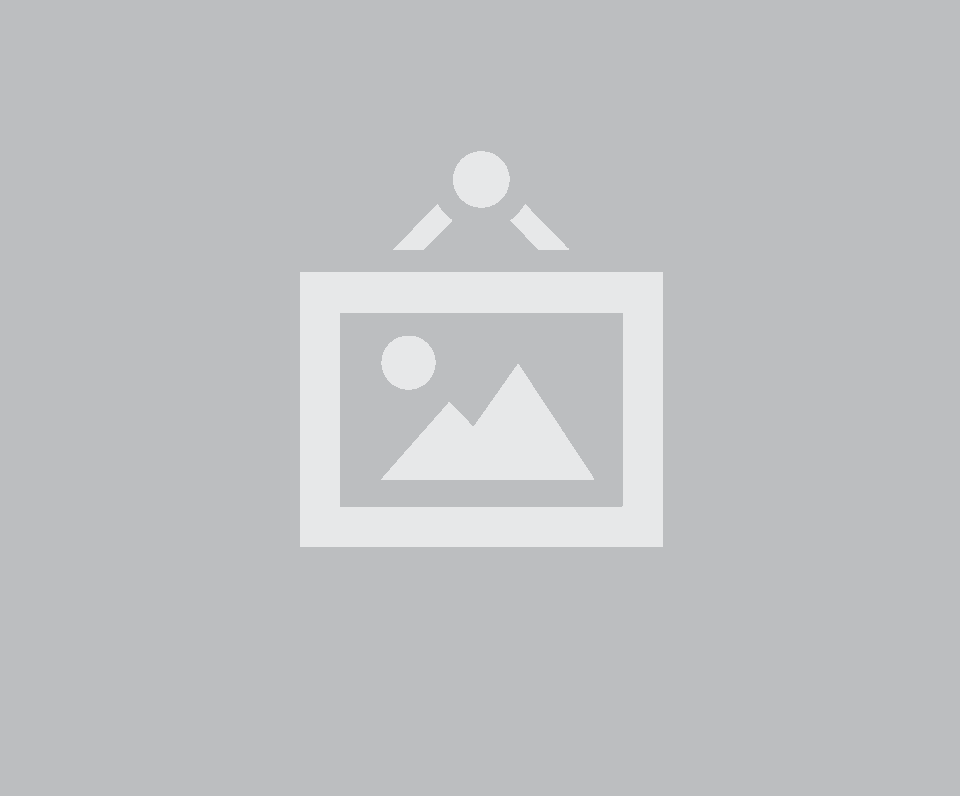
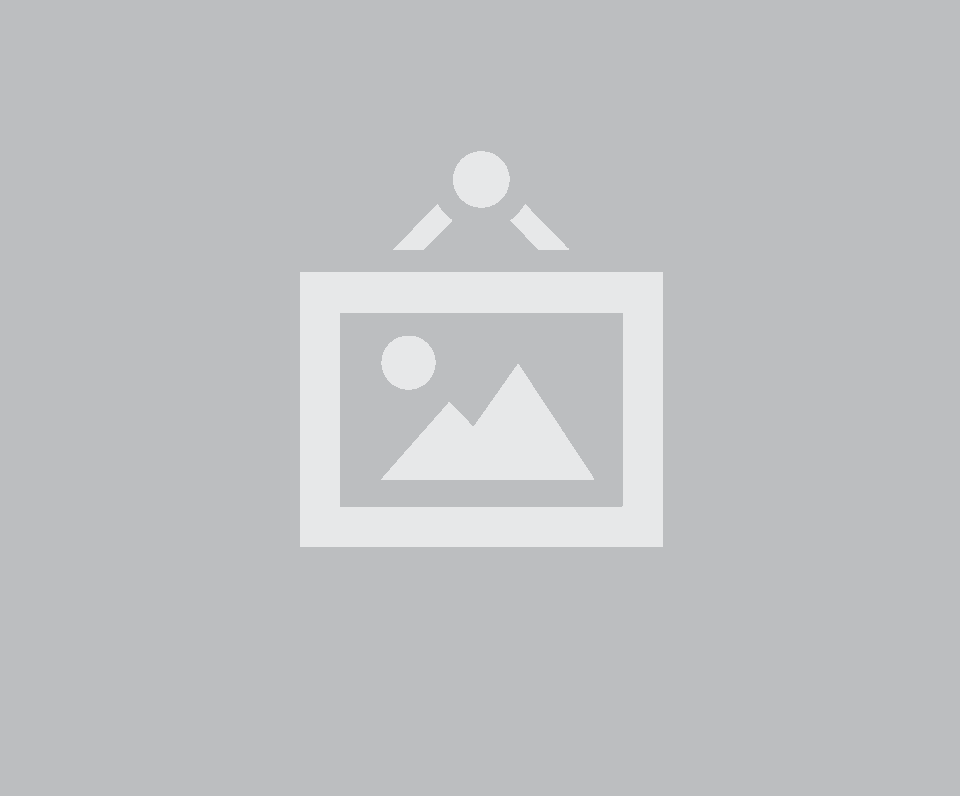
Static Animations
Animations that do not have an in or out animation defined, will maintain their current visibility after running
Looping
Animation loops can be controlled by setting looping. Callbacks will occur after each loop cycle
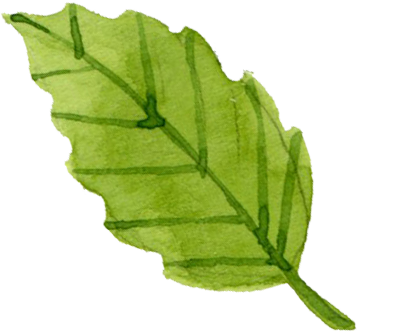
Introduction
UI Transitions provide a wrapper for using CSS transitions in Javascript providing cross-browser callbacks, advanced queuing, and feature detection.
Transitions are loosely coupled with other ui modules like dropdowns and modals to provide element transitions
Types
Transitions are separated into three categories. Inward transitions, outward transitions, and static transitions. These three categories determine the visibility of the element after the animation completes.
Usage
Initializing
If a transition is called without any arguments all default settings will be used.
Passing in settings
Transitions use similar argument shorthand as animate. You can specify callback functions, animation duration, and other settings using the same arguments. Durations can be specified as strings with CSS shorthand, or with numbers.
Display Type
Animations can be used on any display type not just block level elements. For example you can animate a button while preserving its inline-block
status.
Queuing animations
Animations called in sequence will be queued. Any queued animation will automatically determine the transition direction if none is specified.
Stopping Animations
Animations can be stopped using three methods. stop
will end the current animation, stop all
will end animation and remove queued animations, and clear queue
will continue the current playback but remove queued animations.
Hide/Show Without Transition
You can use transitions show
and hide
behavior to hide content without animation. This will preserve the display type, like flex
or table-cell
of an element just like animations.
John Lilki | jhlilk22@yahoo.com | No |
Jamie Harington | jamieharingonton@yahoo.com | Yes |
Jill Lewis | jilsewris22@yahoo.com | Yes |
Behavior
All the following behaviors can be called using the syntax:
Behavior | Description |
---|---|
stop | Stop current animation and preserve queue |
stop all | Stop current animation and queued animations |
clear queue | Clears all queued animations |
show | Stop current animation and show element |
hide | Stop current animation and hide element |
toggle | Toggles between hide and show |
force repaint | Forces reflow using a more expensive but stable method |
repaint | Triggers reflow on element |
reset | Resets all conditions changes during transition |
set looping | Enables animation looping |
remove looping | Removes looping state from element |
disable | Adds disabled state (stops ability to animate) |
enable | Removes disabled state |
set duration(duration) | Modifies element animation duration |
save conditions | Saves all class names and styles to cache to be retrieved after animation |
restore conditions | Adds back cached names and styles to element |
is visible | Returns whether element is currently visible |
is animating | Returns whether transition is currently occurring |
is looping | Returns whether animation looping is set |
is supported | Returns whether animations are supported |
destroy | Destroys instance and removes all events |
Transition Settings
Form settings modify the transition behavior
Setting | Default | Description |
---|---|---|
animation | fade | Named animation event to used. Must be defined in CSS. |
interval | 0 | Interval in MS between each elements transition |
reverse | auto | When an interval is specified, sets order of animations. auto reverses only animations that are hiding. |
displayType | false | Specify the final display type (block, inline-block etc) so that it doesn't have to be calculated. |
duration | false | Duration of the CSS transition animation |
useFailSafe | true | If enabled a timeout will be added to ensure animationend callback occurs even if element is hidden |
failSafeDelay | 100 | Delay in ms for fail safe |
allowRepeats | false | If enabled will allow same animation to be queued while it is already occurring |
queue | true | Whether to automatically queue animation if another is occurring |
skipInlineHidden | false | Whether initially inline hidden objects should be skipped for transition. Useful, if you do the transition for child objects also, but want to have inline hidden children (defined by style="display:none;" ) still kept hidden while the parent gets animated. Accordion uses this. |
Callbacks
Callbacks specify a function to occur after a specific behavior.
Setting | Context | Description |
---|---|---|
onShow | transitioned element | Callback on each transition that changes visibility to shown. Returning false from this callback will cancel the transition from showing. |
onBeforeShow(showFunction) | transitioned element | Callback right before the show transition should start. The showFunction parameter has to be called inside the callback to trigger the transition show |
onVisible | transitioned element | Callback once the show transition has finished. |
onHide | transitioned element | Callback on each transition that changes visibility to hidden. Returning false from this callback will cancel the transition from hiding. |
onBeforeHide(hideFunction) | transitioned element | Callback right before the hide transition should start. The hideFunction parameter has to be called inside the callback to trigger the transition hide |
onHidden | transitioned element | Callback once the transition hide has finished. |
onStart | transitioned element | Callback on animation start, useful for queued animations |
onComplete | transitioned element | Callback on each transition complete |
DOM Settings
DOM settings specify how this module should interface with the DOM
Setting | Default | Description |
---|---|---|
namespace | transition | Event namespace. Makes sure module teardown does not effect other events attached to an element. |
className |
className : {
animating : 'animating',
disabled : 'disabled',
hidden : 'hidden',
inward : 'in',
loading : 'loading',
looping : 'looping',
outward : 'out',
transition : 'transition',
visible : 'visible'
}
|
Class names used to attach style to state |
Debug Settings
Debug settings controls debug output to the console
Setting | Default | Description |
---|---|---|
name | Transition | Name used in debug logs |
silent | false | Silences all console output including error messages, regardless of other debug settings. |
debug | false | Provides standard debug output to console |
performance | true | Provides standard debug output to console |
verbose | false | Provides ancillary debug output to console |
errors |
errors : {
noAnimation : 'There is no CSS animation matching the one you specified.',
method : 'The method you called is not defined',
support : 'This browser does not support CSS animations'
}
|