Types
Javascript
Popup
An element can specify popup content to appear
Titled
An element can specify popup content with a title



HTML
An element can specify HTML for a popup
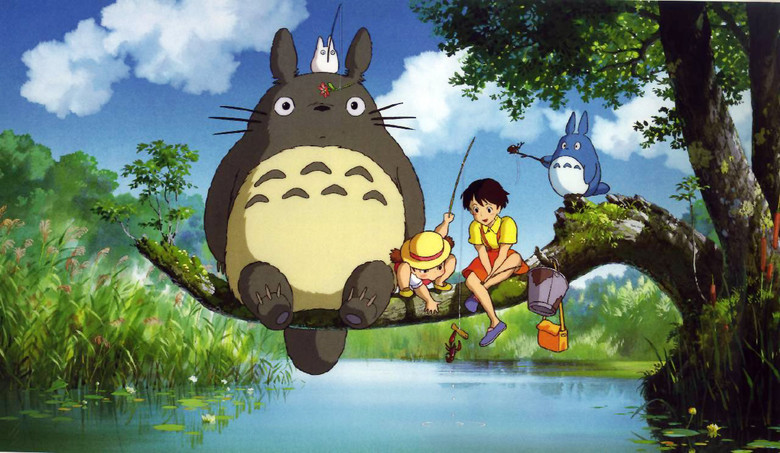
Pre-Existing
An element can display a popup that is already included in the page
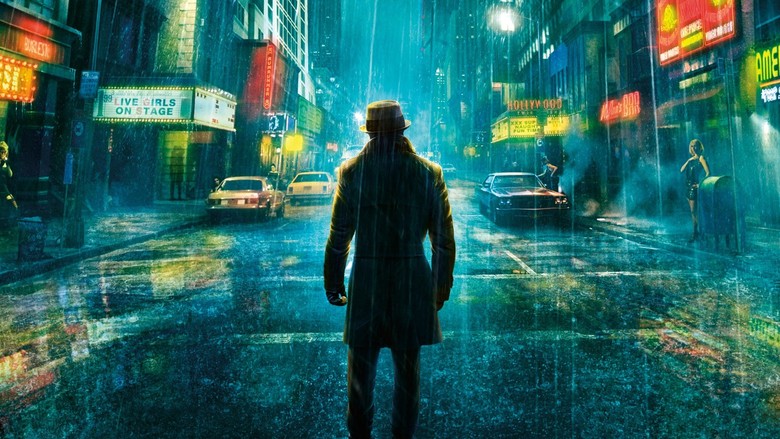
No Javascript
Tooltip
An element can specify a simple tooltip that can appear without javascript via the data-tooltip
attribute. If the tooltip should be shown inverted, also add the data-inverted
attribute.
Tooltip Position
A tooltip can be shown at a specific position relative to the element via the data-position
attribute.
Tooltip Size
A tooltip can vary in size via the data-variation
attribute.
Basic Tooltip
A tooltip can provide more basic formatting
Multiline Tooltip
A tooltip can show multiple lines via predefined line breaks in the HTML code and adding multiline
to the data-variation
attribute.
Visible Tooltip
A tooltip can be shown visible all the time by adding visible
to the data-variation
attribute.
Colored Tooltip
A tooltip can have different colors via the data-variation
attribute.
States
Loading
A popup may show its content is being loaded
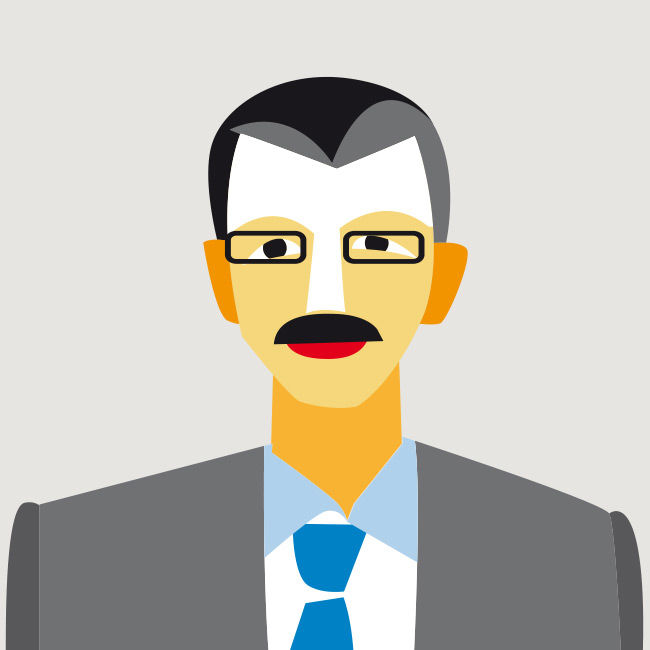
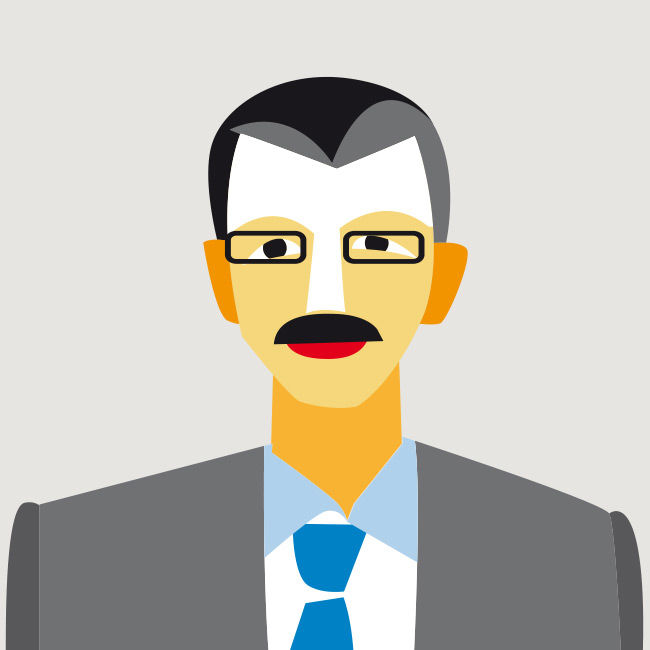
The loader inherits the color of the popup
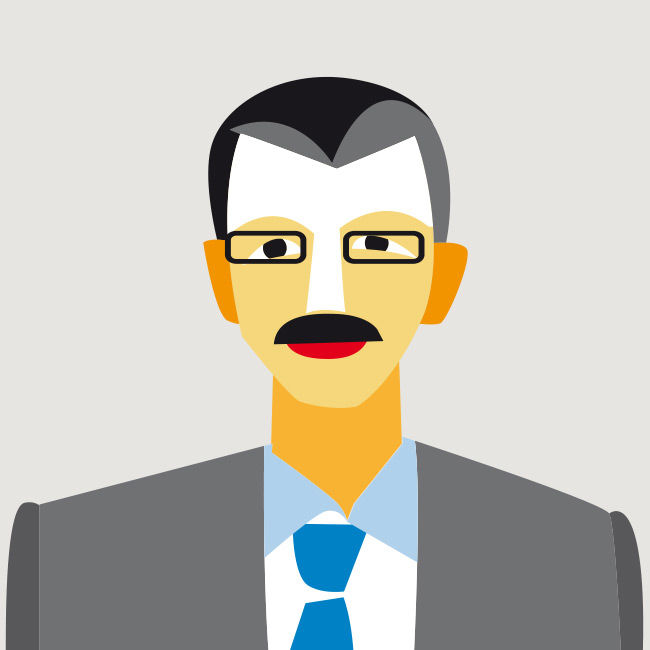
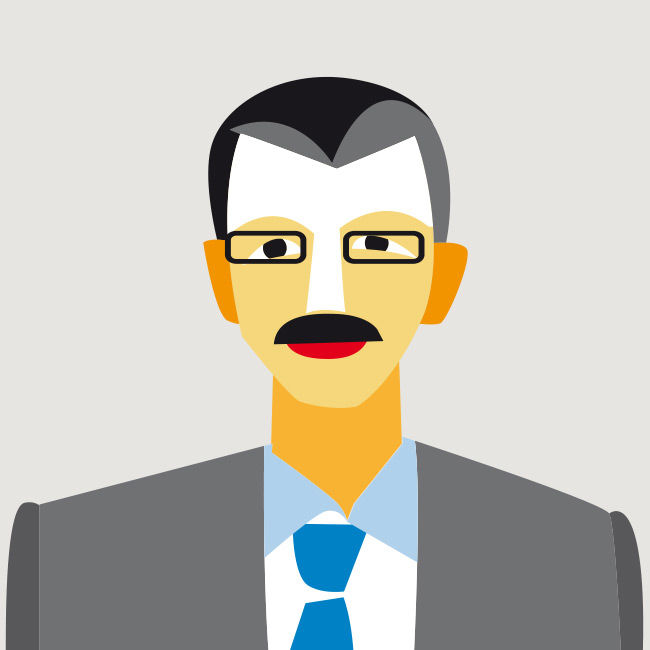
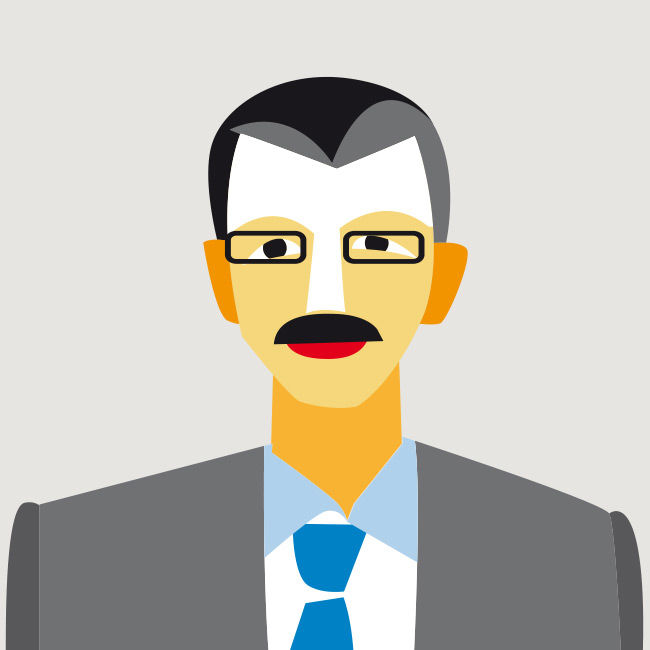
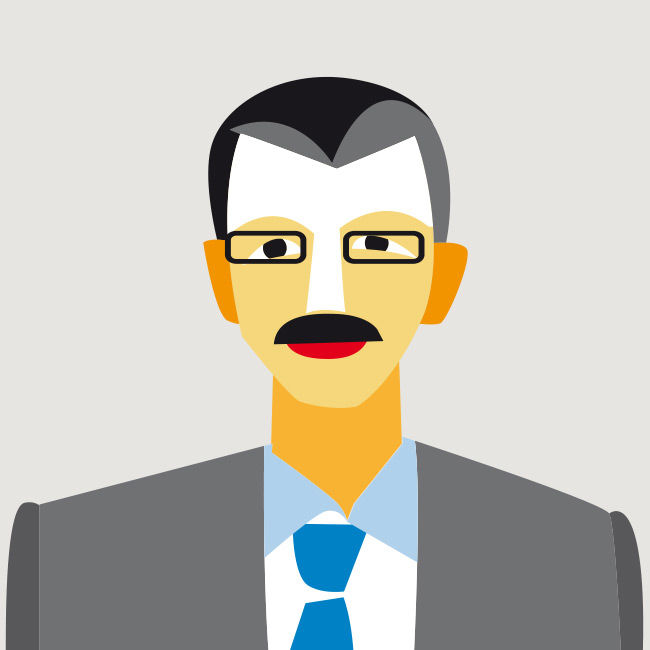
Variations
Basic
A popup can provide more basic formatting
Width
A popup can be extra wide to allow for longer content
Fluid
A fluid popup will take up the entire width of its offset container
Size
A popup can vary in size
Flowing
A popup can have no maximum width and continue to flow to fit its content
Basic Plan
2 projects, $10 a month
Business Plan
5 projects, $20 a month
Premium Plan
8 projects, $25 a month
Inverted
A popup can have its colors inverted
Colored
A popup can have different colors
Usage
Initializing A Popup
A popup is initialized on an activating element
Using a Pre-existing Popup
Using a pre-existing popup allows for you to include complex HTML inside your popup.
If you include your popup on page load as an adjacent sibling element to your activating element it can be found automatically.
To instruct popup to look inline for your popup element you can initialize it with the inline
parameter
Using a Pre-existing Popup Anywhere
If you cannot include your popup element as a sibling element, you can specify a custom selector to retrieve your popup
Specifying Content In Metadata
Frequently used settings like, title, content, HTML, or offset or variation, can be included in HTML metadata
Specifying Content In Javascript
Behavior
All the following behaviors can be called using the syntax:
Behavior | Description |
---|---|
show | Shows popup |
hide | Hides popup |
hide all | Hides all visible pop ups on the page |
get popup | Returns current popup dom element |
change content(html) | Changes current popup content |
toggle | Toggles visibility of popup |
is visible | Returns whether popup is visible |
is hidden | Returns whether popup is hidden |
exists | Returns whether popup is created and inserted into the page |
reposition | Adjusts popup when content size changes (only necessary for centered popups) |
set position(position) | Repositions a popup |
destroy | Removes popup from the page and removes all events |
remove popup | Removes popup from the page |
set loading | Sets popup into loading state displaying a loading spinner and disabled content |
remove loading | Removes loading state from popup |
Examples
Specifying Popup Boundaries
Popups now include a new setting boundary
that let you specify that a popup should not escape the boundary of another section. This can be useful in complex paned layouts
Additionally popups can now specify a scroll context, to allow for scroll containers other than window to cause a clicked popup to hide on scroll.
Normally this popup would open in the default position top center
but because this would escape the boundaries of the segment it will search other available positions until it can find one to place the popup while staying inside the segment
Wide Popup Menu
An easier way to display complex content, like a wide popup menu is to have the popup content as a pre- existing part of your page's HTML.
Using the setting inline: true
will let Fomantic know to display the next sibling ui popup
element after the activator.
Tweaking settings like the delay for show, and hide, and making the menu hoverable will help it function more like a dropdown menu
Specifying a selector for a popup
If its not possible to include the popup content as the next sibling, you can also specify a custom selector to help link the popup contents to its activator.
Specifying a trigger event
A popup trigger event can be specified
Target Element
A popup can specify a different target element than itself to show a popup
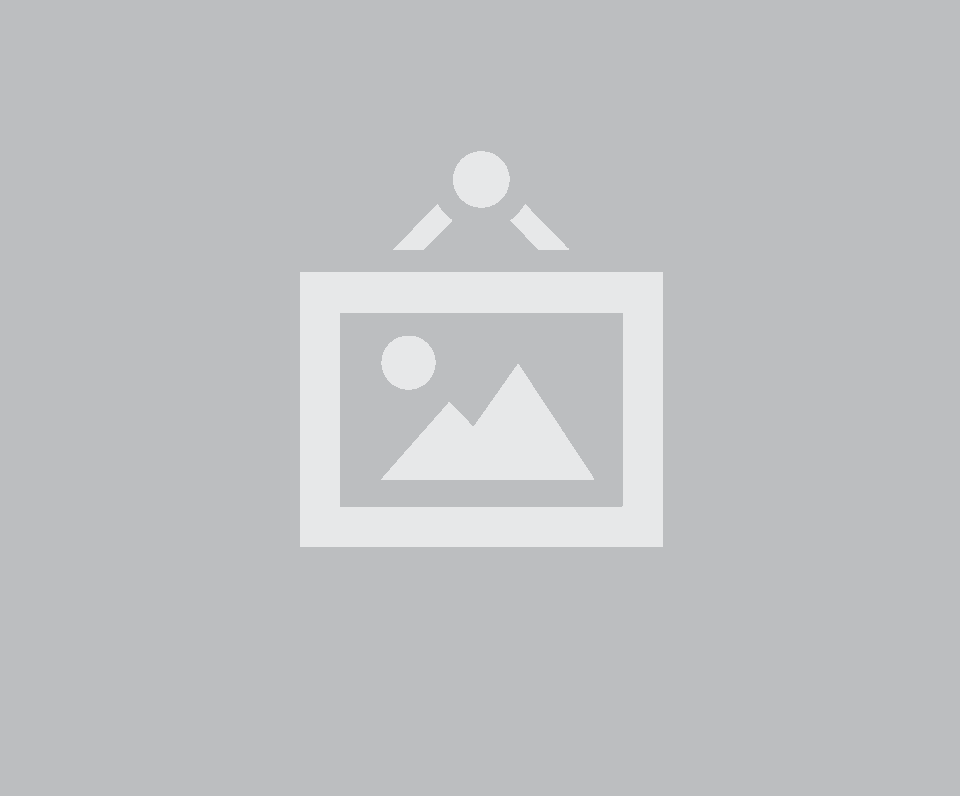
Inline or relative to page
A popup can either be inserted directly after an element, or added as a child element to the page's body
.
Positioning
A popup can be positioned to any side of an element. If space is not available, it will automatically search for a similar alternative position to use.
Specifying an offset
A popup position can be adjusted manually by specifying an offset property using data-offset="value"
or via offset
setting
Transitions
A popup can use any named ui transition.
Home
Popup Settings
Settings to configure popup behavior
Setting | Default | Description |
---|---|---|
popup | false | Can specify a DOM element that should be used as the popup. This is useful for including a pre-formatted popup. |
exclusive | false | Whether all other popups should be hidden when this popup is opened |
movePopup | true | Whether to move popup to same offset container as target element when popup already exists on the page. Using a popup inside of an element without overflow:visible , like a sidebar, may require you to set this to false |
observeChanges | true | Whether popup should attach mutationObservers to automatically run destroy when the element is removed from the page's DOM. |
boundary | window | When the popup surpasses the boundary of this element, it will attempt to find another display position. |
context | body | Selector or jquery object specifying where the popup should be created. |
scrollContext | window | Will automatically hide a popup on scroll event in this context |
jitter | 2 | Number of pixels that a popup is allowed to appear outside the boundaries of its context. This allows for permissible rounding errors when an element is against the edge of its context . |
position | top left | Position that the popup should appear |
forcePosition | false | If given position should be used, regardless if popup fits |
inline | false | If a popup is inline it will be created next to current element, allowing for local css rules to apply. It will not be removed from the DOM after being hidden. Otherwise popups will appended to body and removed after being hidden. |
preserve | false | Whether popup contents should be preserved in the page after being hidden, allowing it to re-appear slightly faster on subsequent loads. |
prefer | opposite | Can be set to adjacent or opposite to prefer adjacent or opposite position if popup cannot fit on screen |
lastResort | false | When set to false , a popup will not appear and produce an error message if it cannot entirely fit on page. Setting this to a position like, right center forces the popup to use this position as a last resort even if it is partially offstage. Setting this to true will use the last attempted position. |
on | hover | Event used to trigger popup. Can be either focus, click, hover, or manual. Manual popups must be triggered with $('.element').popup('show'); |
delay |
delay: {
show: 50,
hide: 70
}
|
Delay in milliseconds before showing or hiding a popup on hover or focus |
transition | scale | Named transition to use when animating menu in and out. Alternatively you can provide an object to set individual values for hide/show transitions as well as hide/show duration
{
showMethod : 'fade',
showDuration : 200,
hideMethod : 'zoom',
hideDuration : 500,
}
|
duration | 200 | Duration of animation events. The value will be ignored when individual hide/show duration values are provided via the transition setting |
arrowPixelsFromEdge | 20 | When a target element is less than 2x this amount, the popup will appear with the arrow centered on the target element, instead of with the popup edge matching the target's edge. |
setFluidWidth | true | Whether popup should set fluid popup variation width on load to avoid width: 100% including padding |
hoverable | false | Whether popup should not close on hover (useful for popup navigation menus) |
closable | true | Whether popup should hide when clicking on the page, auto only hides for popups without on: 'hover' |
addTouchEvents | true | When using on: 'hover' whether touchstart events should be added to allow the popup to be triggered |
hideOnScroll | auto | Whether popup should hide on scroll or touchmove, auto only hides for popups without on: 'click' .Set this to false to prevent mobile browsers from closing popups when you tap inside input fields. |
target | false | If a selector or jQuery object is specified this allows the popup to be positioned relative to that element. |
distanceAway | 0 | Offset for distance of popup from element |
offset | 0 | Offset in pixels from calculated position |
maxSearchDepth | 15 | Number of iterations before giving up search for popup position when a popup cannot fit on screen |
Callbacks
Callbacks specify a function to occur after a specific behavior.
Parameters | Context | Description | |
---|---|---|---|
onCreate | $module | $popup | Callback on popup element creation, with created popup |
onRemove | $module | $popup | Callback immediately before Popup is removed from DOM |
onShow | $module | $popup | Callback before popup is shown. Returning false from this callback will cancel the popup from showing. |
onVisible | $module | $popup | Callback after popup is shown |
onHide | $module | $popup | Callback before popup is hidden. Returning false from this callback will cancel the popup from hiding. |
onHidden | $module | $popup | Callback after popup is hidden |
onUnplaceable | $module | $popup | Callback after popup cannot be placed on screen |
Content Settings
Settings to specify popup contents
Setting | Description |
---|---|
variation | Popup variation to use, can use multiple variations with a space delimiter |
content | Content to display |
title | Title to display alongside content |
html | HTML content to display instead of preformatted title and content |
DOM Settings
DOM settings specify how this module should interface with the DOM
Setting | Default | Description |
---|---|---|
namespace | popup | Event namespace. Makes sure module teardown does not effect other events attached to an element. |
selector |
selector : {
popup : '.ui.popup'
}
|
DOM Selectors used internally |
metadata |
metadata: {
activator : 'activator',
content : 'content',
html : 'html',
offset : 'offset',
position : 'position',
title : 'title',
variation : 'variation'
}
|
HTML Data attributes used to store data |
className |
className : {
active : 'active',
basic : 'basic',
animating : 'animating',
dropdown : 'dropdown',
invisible : 'invisible',
fluid : 'fluid',
loading : 'loading',
popup : 'ui popup',
position : 'top left center bottom right',
visible : 'visible',
popupVisible: 'visible'
}
|
Class names used to attach style to state |
Debug Settings
Debug settings controls debug output to the console
Setting | Default | Description |
---|---|---|
name | Popup | Name used in debug logs |
silent | false | Silences all console output including error messages, regardless of other debug settings. |
debug | false | Provides standard debug output to console |
performance | true | Provides standard debug output to console |
verbose | false | Provides ancillary debug output to console |
errors |
error: {
invalidPosition : 'The position you specified is not a valid position',
cannotPlace : 'Popup does not fit within the boundaries of the viewport',
method : 'The method you called is not defined.',
noElement : 'This module requires ui {element}',
notFound : 'The target or popup you specified does not exist on the page'
}
|